STOP THE FRAME DROP - Texture (AsyncDisplayKit)
The last couple of days I've been working with Texture (AsyncDisplayKit), it's a cool framework that basically makes your app run more efficiently. It's all about the performance gains. Texture works with nodes which are thread-safe. Unlike views, which can only be used on the main thread.
Today I will show you how you can create a really efficient tableView with Texture, and you'll clearly see the performance gains from it. Since Texture keeps expensive UI operations off the main thread it is available to respond to user interactions at any given time.
[/bscolumns][bscolumns class="one_third"]
[/bscolumns][bscolumns class="one_third_last"][/bscolumns][bscolumns class="clear"][/bscolumns]
You can clearly see that the the first image moves smoother than the second one. It's because all images and text fields are rendered in the background instead of the main thread.
Texture also makes it easy to add placeholders. The reason you'll want to use placeholders is that it can take a few milliseconds to load the actual views from the background thread.
Below you can see the difference between the two:
[bscolumns class="one_third"]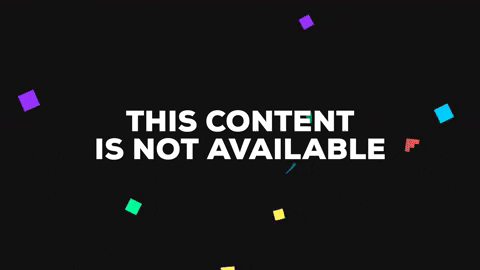
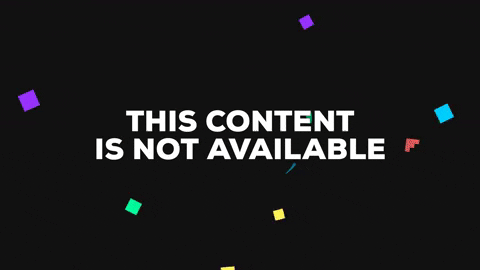
Installation
To install it you can just use CocoaPods: [code lang="swift"]pod "Texture"[/code] Or Carthage: [code lang="swift"]Github “texturegroup/texture”[/code]Usage
First, let's import the dependency: [code lang="swift"] import AsyncDisplayKit [/code] After that we will create the class itself: [code lang="swift"] class NodeViewController: ASViewController<ASDisplayNode>, ASTableDataSource, ASTableDelegate { var tableNode: ASTableNode { return node as! ASTableNode } init() { super.init(node: ASTableNode()) self.tableNode.delegate = self self.tableNode.dataSource = self } required init?(coder aDecoder: NSCoder) { fatalError("storyboards are incompatible with truth and beauty") } [/code] There are a few big changes here that probably going to need some explanation:- Instead of creating a UIViewController class we will create an ASViewController class which is a subclass of UIViewController. It does a few things automatically for you like if the view controller goes off screen it will automatically reduce the size of the fetch data and display ranges of any of its children.
- A UITableView doesn't exist anymore, we will use an ASTableNode which uses ASTableDataSource and ASTableDelegate. They are very similar to UITableViewDataSource and UITableViewDelegate.
- Instead of an UIImageView we will use an ASImageNode and instead of an UITextField we will use an ASTextNode.
- Instead of addSubview we will use addSubnode.